Editing class properties in different places in the editor
When developing with Unreal, it is common for programmers to implement properties on Actors or other objects in C++, and make them visible in the editor for designer use. However, sometimes, it makes sense to view a property, or make it editable, but only on the object’s default state. Sometimes, the property should only be modifiable at runtime with the default specified in C++. Fortunately, there are some specifiers that can help us restrict when a property is available.
How to do it…
- Create a new Actor class in the editor called PropertySpecifierActor:
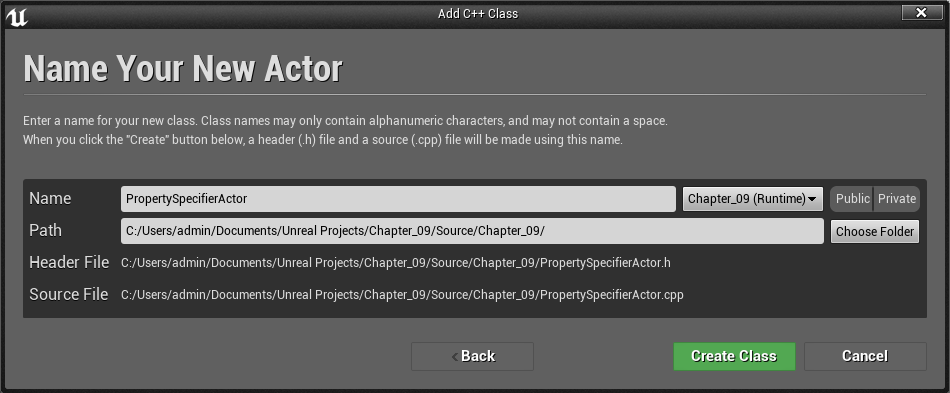
- Add the following property definitions to the class:
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "PropertySpecifierActor.generated.h"
UCLASS()
class CHAPTER_09_API APropertySpecifierActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
APropertySpecifierActor();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
// Property Specifiers
UPROPERTY(EditDefaultsOnly)
bool EditDefaultsOnly;
UPROPERTY(EditInstanceOnly)
bool EditInstanceOnly;
UPROPERTY(EditAnywhere)
bool EditAnywhere;
UPROPERTY(VisibleDefaultsOnly)
bool VisibleDefaultsOnly;
UPROPERTY(VisibleInstanceOnly)
bool VisibleInstanceOnly;
UPROPERTY(VisibleAnywhere)
bool VisibleAnywhere;
};
- Perform a Save, Compile your code, and launch the editor.
- Create a new blueprint based on the class.
- Open the blueprint and look at the Class Defaults section:
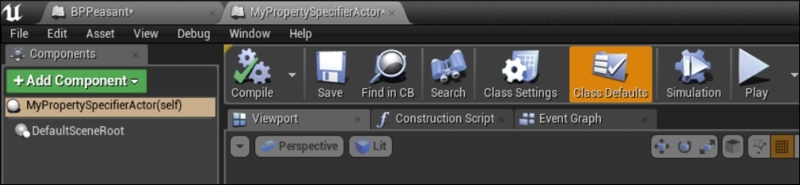
- Note which properties are editable and visible under the Property Specifier Actor section:
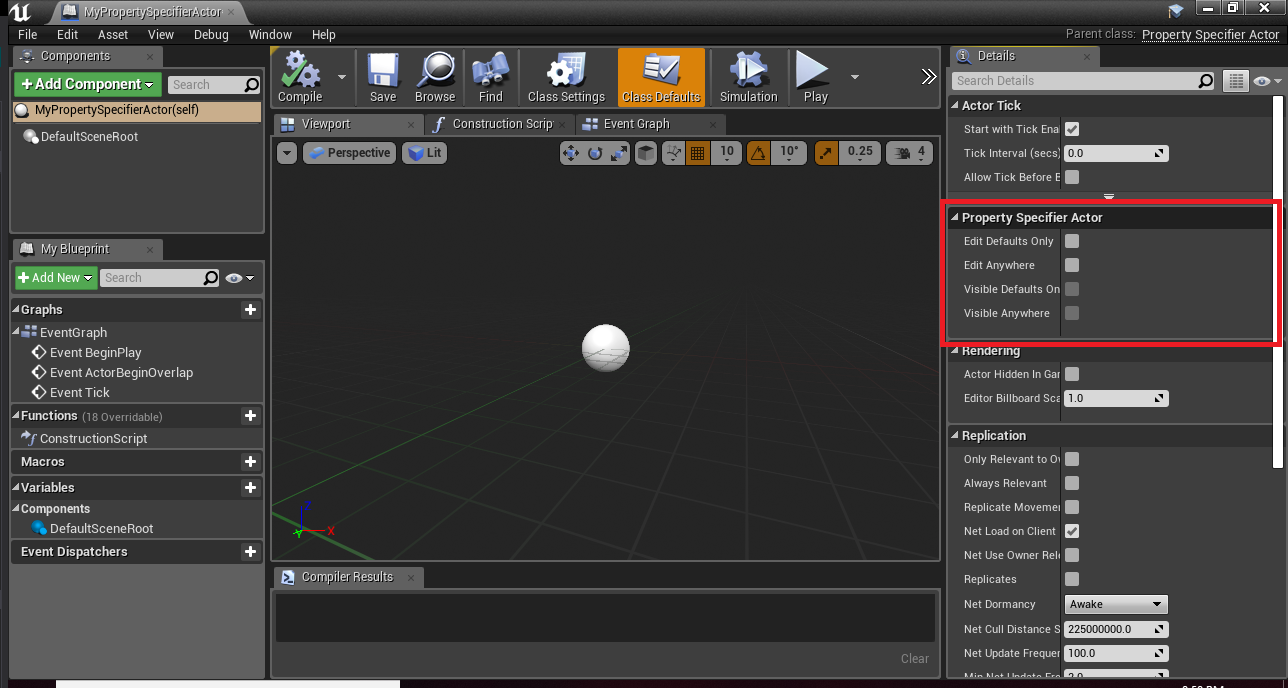
Location of the Property Specifier Actor
- Place instances in the level and view their Details panels:

- Note that a different set of properties is editable.
How it works…
When specifying UPROPERTY, we can indicate where we want that value to be available inside the Unreal editor.
Visible* prefixes indicate that the value is viewable in the Details panel for the indicated object. The value won’t be editable, however.
This doesn’t mean that the variable is a const qualifier; however, native code can change the value, for instance.
Edit* prefixes indicate that the property can be altered within the Details panels inside the editor.
InstanceOnly as a suffix indicates that the property will only be displayed in the Details panels for instances of your class that have been placed into the game. They won’t be visible in the Class Defaults section of the Blueprint editor, for example.
DefaultsOnly is the inverse of InstanceOnly – UPROPERTY will only display in the Class Defaults section, and can’t be viewed on individual instances within the level.
The suffix Anywhere is the combination of the two previous suffixes – the UPROPERTY will be visible in all the Details panels that inspect either the object’s defaults or a particular instance in the level.As we mentioned previously, if you are interested in learning more about Property Specifiers, check out the following link: https://docs.unrealengine.com/en-us/Programming/UnrealArchitecture/Reference/Properties/Specifiers.
See also
- This recipe makes the property in question visible in the inspector, but doesn’t allow the property to be referenced in the actual Blueprint Event Graph. See the following recipe for a description of how to make that possible.
Post Link: Editing class properties in different places in the editor