Making properties accessible in the Blueprint editor graph
The specifiers we mentioned in the previous recipe are all well and good, but they only control the visibility of UPROPERTY in the Details panel. By default, even with those specifiers used appropriately, UPROPERTY won’t be viewable or accessible in the actual editor graph for use at runtime.Other specifiers, which can optionally be used in conjunction with the ones in the previous recipe so that you can interact with properties in the Event Graph.
How to do it…
- Create a new Actor class called BlueprintPropertyActor using the editor wizard:
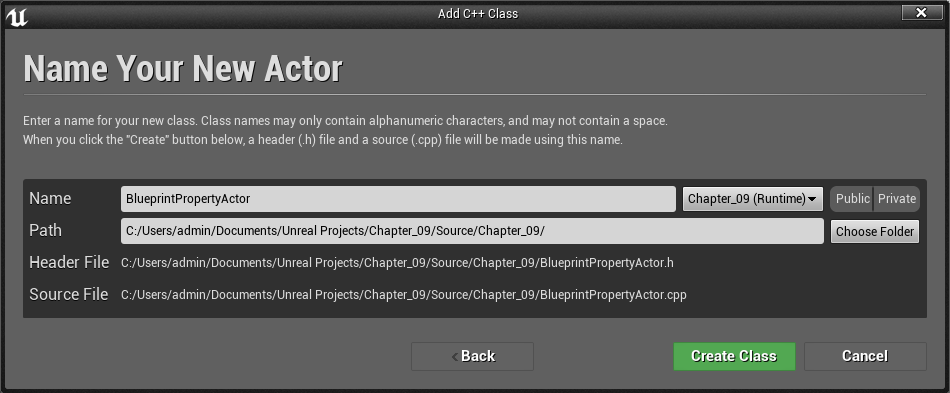
- Add the following UPROPERTY to the class using Visual Studio:
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "BlueprintPropertyActor.generated.h"
UCLASS()
class CHAPTER_09_API ABlueprintPropertyActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
ABlueprintPropertyActor();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
UPROPERTY(BlueprintReadWrite, Category = Cookbook)
bool ReadWriteProperty;
UPROPERTY(BlueprintReadOnly, Category = Cookbook)
bool ReadOnlyProperty;
};
- Perform a Save, Compile your project, and start the editor.
- Create a Blueprint class based on your BlueprintPropertyActor and open its graph.
- From the My Blueprint panel, click on the eye icon to the right of the Search bar. From there, select Show Inherited Variables:
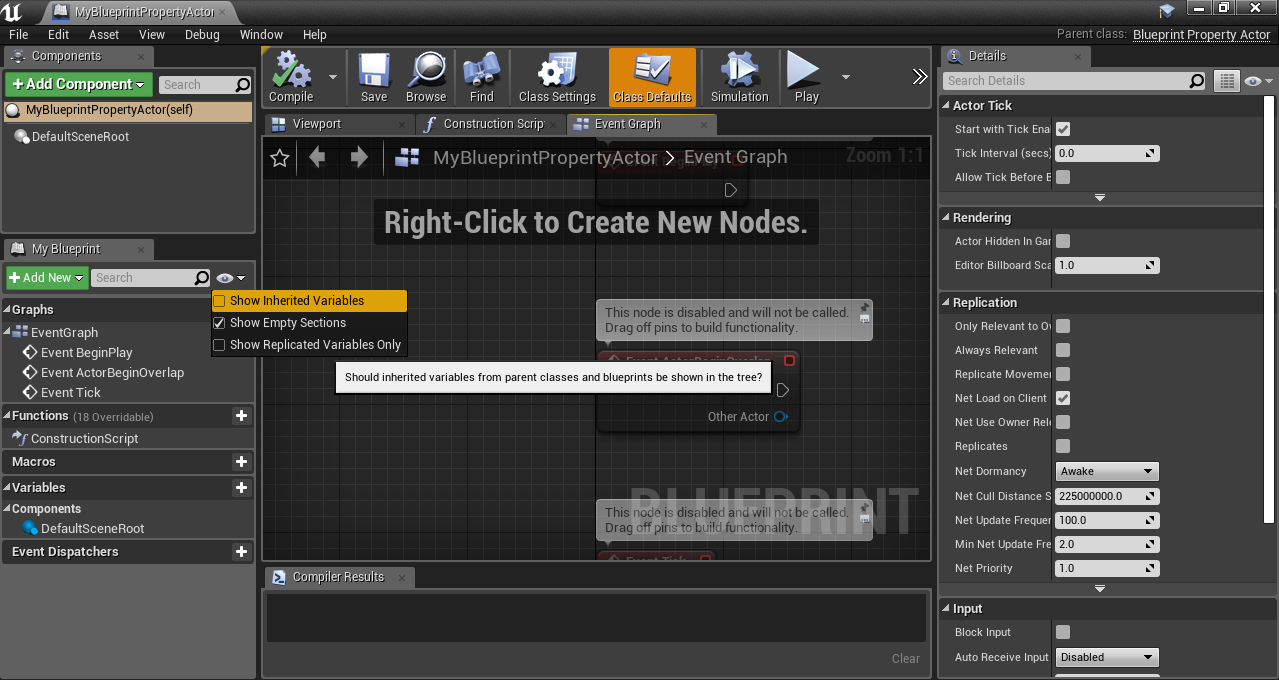
- Verify that the properties are visible under the Cookbook category in the Variables section of the My Blueprint panel:
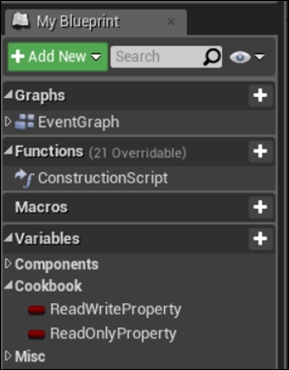
- Left-click and drag the ReadWriteProperty variable into the Event Graph. Then select Get ReadWriteProperty:
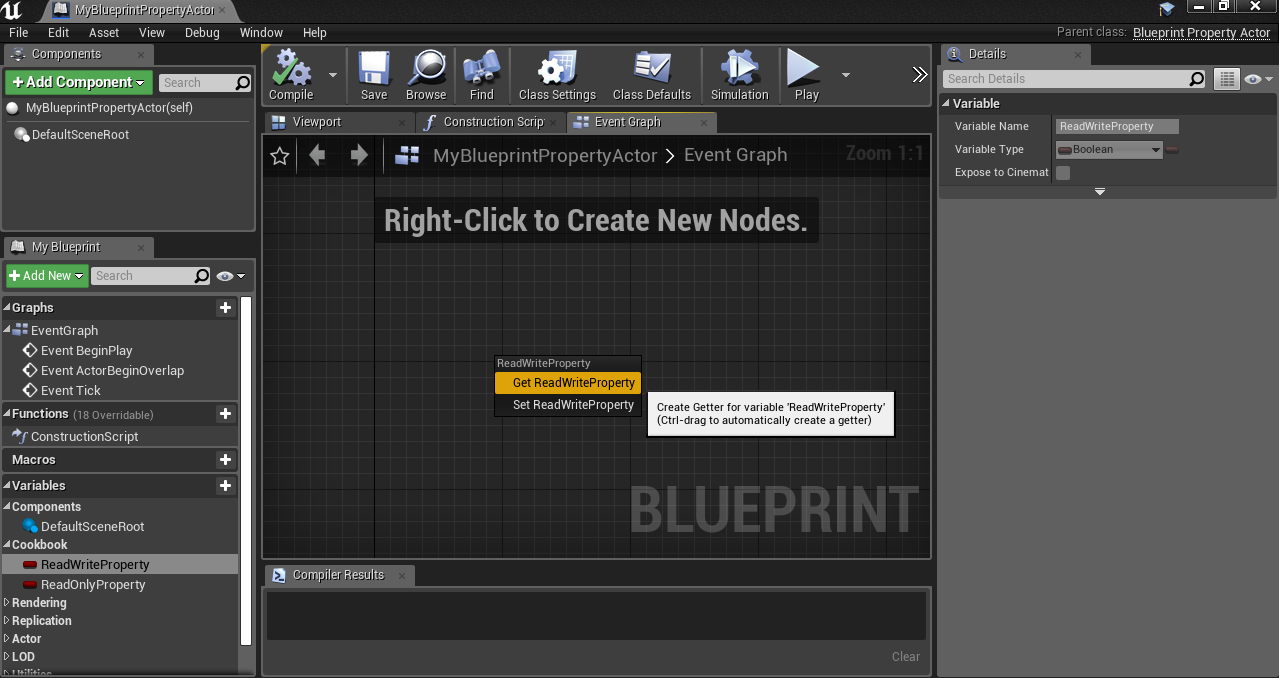
- Repeat the previous step, but instead select Set ReadWriteProperty.
- Drag the ReadOnly property into the graph and note that the SET node is disabled:

How it works…
BlueprintReadWrite as a UPROPERTY specifier indicates to the Unreal Header Tool that the property should have both Get and Set operations exposed for use in Blueprints.
BlueprintReadOnly is, as the name implies, a specifier that only allows Blueprint to retrieve the value of the property; never set it.
BlueprintReadOnly can be useful when a property is set by native code, but should be accessible within Blueprint.
It should be noted that BlueprintReadWrite and BlueprintReadOnly don’t specify anything about the property being accessible in the Details panels or the My Blueprint section of the editor: these specifiers only control the generation of the getter/setter nodes for use in Blueprint graphs.
Post Link: Making properties accessible in the Blueprint editor graph