Creating new menu entries
The workflow for creating new menu entries is almost identical to that for creating new toolbar buttons, so this recipe will build on the previous one and show you how to add the command created therein to a menu rather than a toolbar.
How to do it…
- Create a new function inside of the FChapter_10EditorModule class, which is in Chapter10_Editor.h:
void AddMenuExtension(FMenuBuilder &builder) { FSlateIcon IconBrush =
FSlateIcon(FEditorStyle::GetStyleSetName(),
"LevelEditor.ViewOptions",
"LevelEditor.ViewOptions.Small"); builder.AddMenuEntry(FCookbookCommands::Get().MyButton); };
- In the implementation file (Chapter_10Editor.cpp), find the following code within the StartupModule function:
EExtension = ToolbarExtender->AddToolBarExtension("Compile", EExtensionHook::Before, CommandList, FToolBarExtensionDelegate::CreateRaw(this, &FChapter_10EditorModule::AddToolbarExtension));
LevelEditorModule.GetToolBarExtensibilityManager()->AddExtender(ToolbarExtender);
- Replace the preceding code with the following:
Extension = ToolbarExtender->AddMenuExtension("LevelEditor", EExtensionHook::Before, CommandList, FMenuExtensionDelegate::CreateRaw(this,&FChapter_10EditorModule::AddMenuExtension));
LevelEditorModule.GetMenuExtensibilityManager()->AddExtender(ToolbarExtender);
- Compile your code and launch the editor.
- Verify that you now have a menu entry under the Window menu that displays the Cookbook window when clicked. If you followed the preceding recipe, you’ll also see the green text listing the UI extension points, including the one we used in this recipe (LevelEditor):
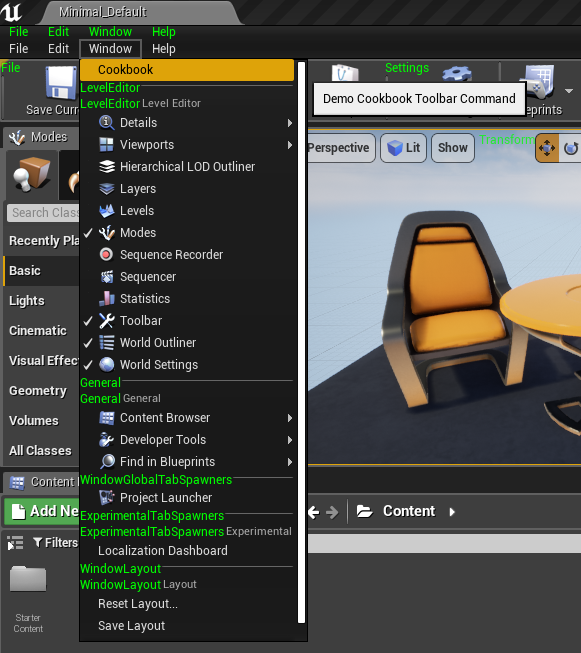
How it works…
You’ll note that ToolbarExtender is of type FExtender rather than FToolbarExtender or FMenuExtender.
By using a generic FExtender class rather than a specific subclass, the framework allows you to create a series of command-function mappings that can be used on either menus or toolbars. The delegate that actually adds the UI controls (in this instance, AddMenuExtension) can link those controls to a subset of commands from your FExtender.
This way, you don’t need to have different TCommands classes for different types of extensions, and you can place the commands into a single central class regardless of where those commands are invoked from the UI.
As a result, the only changes that are required are as follows:
- Swapping calls to AddToolBarExtension with AddMenuExtension
- Creating a function that can be bound to FMenuExtensionDelegate rather than FToolbarExtensionDelegate
- Adding the extender to a Menu Extensibility Manager rather than a Toolbar Extensibility Manager
Post Link: Creating new menu entries