4. Gameplay Classes-1
This chapter looks at some of the important and common gameplay classes you use in almost all projects.
Actor
The Actor class is the base for all placeable and spawnable objects in a game world. Anything that can be dragged and dropped into the world or spawned at runtime is inherited from the Actor class. Actor classes are basic classes that can support translation (position), rotation, and scale (size). Actors can contain any number of Actor Component classes that define how it should move and render. By default, Actors are generic classes and do not have any kind of visual representation. It is up to the component to give it a visual representation. Actor classes also support replication and function calls across the network.
Game Mode Base
Game Mode Base defines the basic rules for your game, such as which actors are allowed, player scores, winning rules, and so forth. It holds information about all the players in the game world. For example, you can define the score required to win a match. Game Mode sets the classes for Pawn, Player Controller, Game State, Player State, Spectator, and more. In a multiplayer game, the Game Mode class only exists on the server, and for the client, it is always null, as if it never exists. This is only applicable to multiplayer games because, in single-player games, there are no clients, only the server.
Game Mode, a subclass of Game Mode Base, is designed toward multiplayer games. It contains default behavior for picking spawn points for each player.
Game State Base
Game State Base defines the current state of the game. Game State classes are spawned by the Game Mode. They are particularly useful in a multiplayer game because Game State classes are replicated to every client, so any change that happens to a replicated value reflects all clients. For example, imagine you have a game mode that requires some number of kills to win the game. Every time the player eliminates another player, it runs a server function that updates the current number of total kills in Game Mode, which is then changed in the game state, and it is updated on all clients. Game State is a subclass of Game State Base.
Game Instance
The Game Instance class is created when you run the game. It exists when the game is running. It is useful for transferring information between levels. For example, imagine in your game that the player finishes a certain challenge. You can store this specific information in Game Instance. When the player returns to the main menu, you can show a message on the screen that says the challenge has been completed. Game Instance is a UObject-based class; it is not replicated to anyone. The server or client is not aware of any other game instances other than their own.Game Instance is set via Project Settings ➤ Maps & Modes ➤ Game Instance class (see Figure 4-1).

The following describes the three main Game classes.
- Game Mode holds authority of the game flow and only exists on the server, which prevents cheating.
- Game State holds the replicated state of the game. It exists on the server and client. Clients can ask the Game State about the current state of the game, and the server can modify the current state of the game, which is replicated to all clients.
- Game Instance is a true persistent class that is guaranteed to be available and accessible from the very beginning of your game until you exit. It is not replicated and exists on both the server and client.
Pawn
Pawn is an Actor-based class that acts as a physical representation of a player or artificial intelligence (AI). Pawns are controlled by a Controller, which is either a Player Controller or an AI Controller. Even though the pawn does not have visual representation by default, it still represents location, rotation, and so forth, within the game world. Pawns can contain their own movement logic, but it’s better done in the Controller. Pawns can be replicated across the network.
Character
Character is extended from the Pawn class to support visual representation by using the Skeletal Mesh Component for animations. It also includes the Capsule Component to simulate movement collisions, and the Character Movement Component, a feature-rich component that includes movements such as walk, run, jump, swim, and fly. It also includes basic networking support, which makes it a first-class choice for bipedal characters. The Character Movement Component is specific to the Character class, so it cannot be used in any other class.
Player Controller
Player Controllers are subclassed from the Controller, which is essentially the brain of the Pawn it is controlling. Based on the context, the Controller is a Player Controller if it is controlled by a human player (and can handle all player inputs) or an AI Controller is controlled by artificial intelligence.
By default, a Controller can only control one Pawn at any given time by calling the Possess() function. It stops controlling by calling the UnPossess() function. A Controller also allows notifications from the Pawn class that it is controlling. In a networked game, the server is aware of all controllers while the client is only aware of its own local controller.
Player State
Player State is a replicated Actor that is available for every player. There is only one player state in a single-player game, but in a multiplayer game, each player has their own player state. Since it is replicated, the server and client are aware of all player states, and all of them can be accessed from the Game State class. This is a good place to store network-relevant information about a player, like score, player name, and so forth.Let’s share a scenario where you can use these classes. When writing this book, battle royale games (Fortnite, PUBG, etc.) are very popular among us gamers, so if you were to create a battle royale game, you could use the following classes.
- Game Mode: This class defines the rules. In this class, you track the number of players that have entered the match, the number that are alive, the number that are dead, and the quitters. This class also tracks and decides other game elements, such as a battle plane (or a battle bus in Fortnite), circle, and so on, and whether the match has started or is waiting to start (warm up).
- Game State: This class tracks the replicated state of the game. For example, when the game mode transitions from prematch (lobby) to a match state (battle plane or bus starting on top of the map), it notifies the game state that the state has changed, and the game state can notify all the clients that match started.
- Game Instance: This class saves information about any objectives or challenges completed in the game so that the player can be rewarded when they return to the main menu.
- Character: The visual representation of the character.
- Pawn: A vehicle or an animal in your game. (Optional)
- Player Controller: This class handles all the input and creates and manages the user interfaces. It can also send RPCs (remote procedure calls) to the server. The server is aware of all player controllers (e.g., if the game has 100 players, the server knows all 100 player controllers), but the client is only aware of its own.
- Player State: This class holds the information related to player-specific states (e.g., the number of players you killed, the amount of ammo you fired, and so forth).
Creating Character Class and Player Controller Class
In this section, you learn how to create a new character class that allows the player to move around in the game world using the Player Controller class. Later in this section, a UMG (Unreal Motion Graphics) widget shows health information to the player.To create a new character class, right-click the Content Browser and select Blueprint Class. You are prompted to pick a parent class for your Blueprint. Select Character from the Common Classes section (see Figure 4-2).
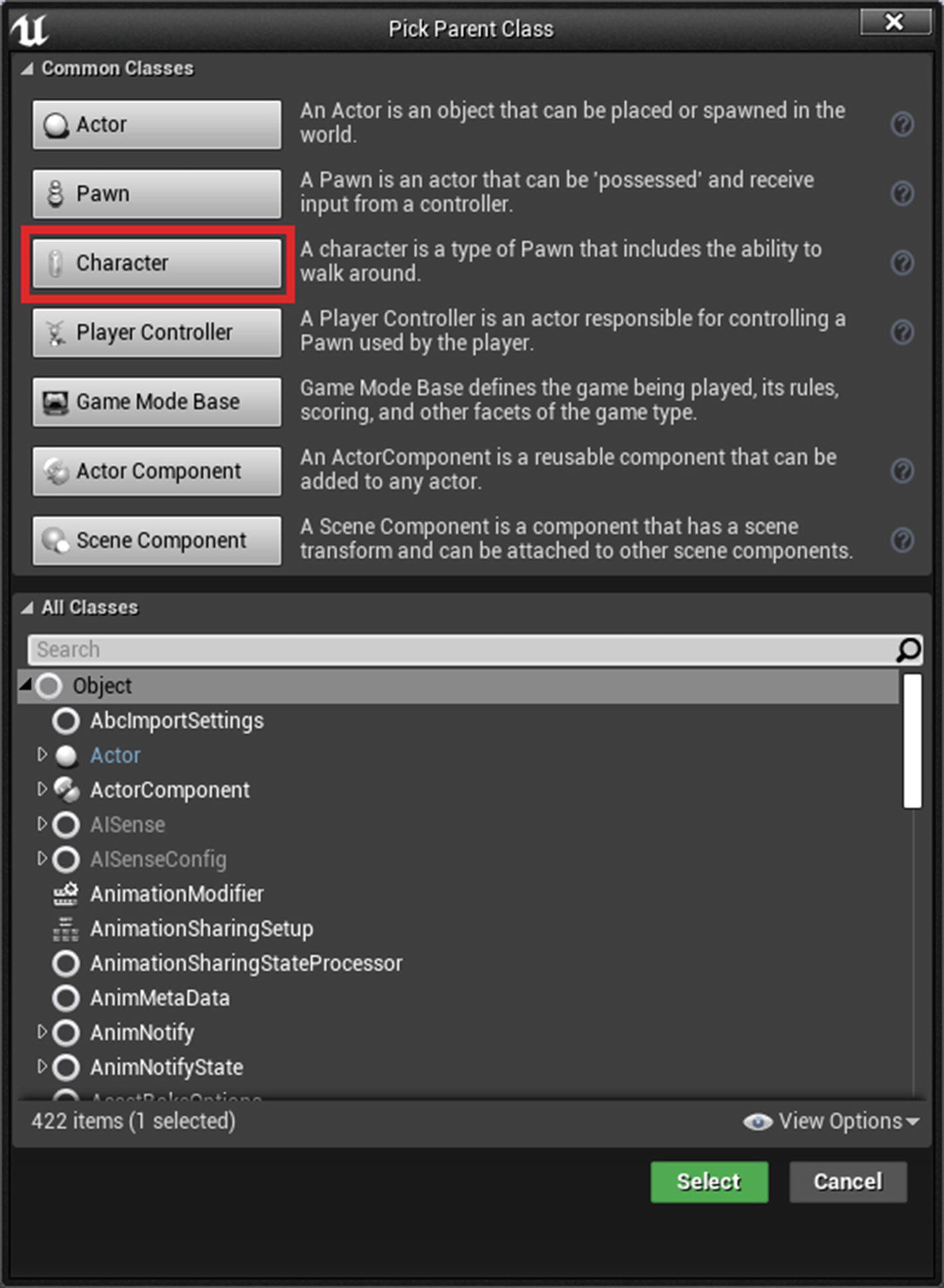
Next, open the Character Blueprint. You see the default Capsule Component, Mesh Component, and Character Movement Component (see Figure 4-3).
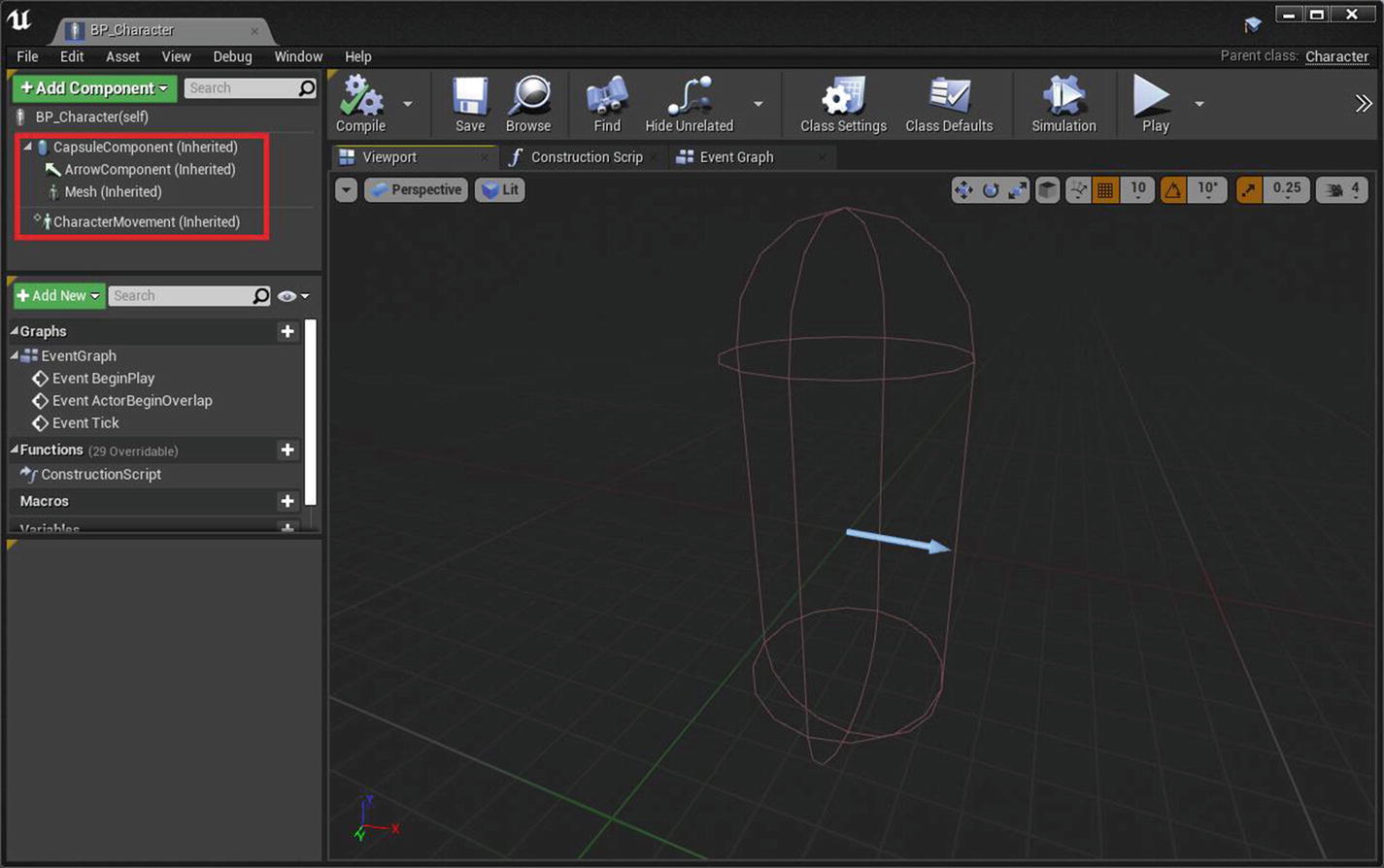
- CapsuleComponent is the actor’s root component. It is responsible for collisions. CharacterMovement is aware of this capsule component and moves it around according to the player input.
- ArrowComponent is an editor-only component that helps indicate which direction the object is facing.
- Mesh is the visual representation of the character and is of the Skeletal Mesh Component type.
- CharacterMovement handles the movement logic of the associated character. This component updates the location and rotation of CapsuleComponent, which makes the character move. It is a highly featured component and includes network support.
We will use the default Mannequin that comes with the Engine as our character. To use it, you must add the Third Person Project to your project. If not yet added, you can do it by clicking the Add New button in the Content Browser and selecting Add Feature or Content Pack (see Figure 4-4).

Post Link: 4. Gameplay Classes-1